For loops
Official definition: https://wiki.python.org/moin/ForLoop
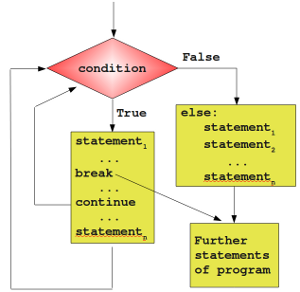
Picture source: https://www.python-course.eu/python3_loops.php
for loops are traditionally used when you have a block of code which you want to repeat a fixed number of times. The Python for statement iterates over the members of a sequence in order, executing the block each time.
Important: The for loop runs for a fixed amount - in this case, 3, while the while loop runs until the loop condition changes; in this example, the condition is the boolean True which will never change, so it could theoretically run forever.
However, like the while loop, the for loop can be made to exit before the given object is finished. This is done using the break statement, which will immediately drop out of the loop and contine execution at the first statement after the block.
fruits = ["apple", "banana", "cherry"] for x in fruits: print(x) if x == "banana": break
apple banana
fruits = ["apple", "banana", "cherry"] for x in fruits: if x == "banana": break print(x)
apple
fruits = ["apple", "banana", "cherry"] for x in fruits: if x == "banana": continue print(x) apple
cherry
string = "Hello World" for x in string: print(x)
H e l l o W o r l d >>>
collection = ['hey', 5, 'd'] for x in collection: print(x)
hey 5 d >>>
Let's look at this:
list_of_lists = [ [1, 2, 3], [4, 5, 6], [7, 8, 9]] for list in list_of_lists: print(list)
[1, 2, 3] [4, 5, 6] [7, 8, 9] >>>
We can use else to print the last item:
list_of_lists = [ [1, 2, 3], [4, 5, 6], [7, 8, 9]] for list in list_of_lists: print(list) else: print(list)
[1, 2, 3] [4, 5, 6] [7, 8, 9] [7, 8, 9] >>>
list_of_lists = [ [1, 2, 3], [4, 5, 6], [7, 8, 9]] for list in list_of_lists: for x in list: print(x)
1 2 3 4 5 6 7 8 9 >>>
Python is structurally strict,
The results of this:
list_of_lists = [ [1, 2, 3], [4, 5, 6], [7, 8, 9]] for list in list_of_lists: for x in list: print(x) else: print('Final x = %d' % (x))
1 2 3 4 5 6 7 8 9 Final x = 9
>>>
While the result of this is:
list_of_lists = [ [1, 2, 3], [4, 5, 6], [7, 8, 9]] for list in list_of_lists: for x in list: print(x) else: print('Final x = %d' % (x))
1 2 3 Final x = 3 4 5 6 Final x = 6 7 8 9 Final x = 9 >>>
If we wanna only print the final results, just keep print command after else and leave the first print command empty.
More about range():
The range() function returns a sequence of numbers, starting from 0 by default, and increments by 1 (by default), and ends at a specified number.
for x in range(6): print(x)
0 1 2 3 4 5
The range() function defaults to 0 as a starting value, however it is possible to specify the starting value by adding a parameter: range(2, 6), which means values from 2 to 6 (but not including 6):
for x in range(2, 6): print(x)
2 3 4 5
By definition, this will give empty results:
for x in range(6, 2): print(x)
The range() function defaults to increment the sequence by 1, however it is possible to specify the increment value by adding a third parameter: range(2, 30, 3):
for x in range(2, 30, 3): print(x)
2 5 8 11 14 17 20 23 26 29
Therefore, this works,
for x in range(6, 2, -1): print(x)
6 5 4 3
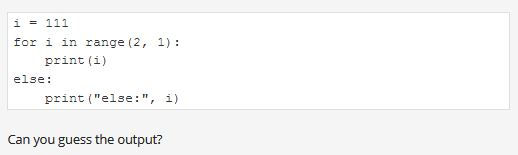
The loop's body won't be executed here at all. Note: we've assigned the i variable before the loop.
When the loop's body isn't executed, the control variable retains the value it had before the loop.
Note: if the control variable doesn't exist before the loop starts, it won't exist when the execution reaches the else branch.