break and continue
- John Fan Zhang
- Jun 16, 2020
- 2 min read
break - exits the loop immediately, and unconditionally ends the loop's operation; the program begins to execute the nearest instruction after the loop's body;
continue - behaves as if the program has suddenly reached the end of the body; the next turn is started and the condition expression is tested immediately.
# break - example print("The break instruction:") for i in range(1, 6): if i == 3: break print("Inside the loop.", i) print("Outside the loop.")
Output:
The break instruction:
Inside the loop. 1
Inside the loop. 2
Outside the loop.
# continue - example print("\nThe continue instruction:") for i in range(1, 6): if i == 3: continue print("Inside the loop.", i) print("Outside the loop.")
Output:
The continue instruction:
Inside the loop. 1
Inside the loop. 2
Inside the loop. 4
Inside the loop. 5
Outside the loop.
largestNumber = -99999999 counter = 0 while True: number = int(input("Enter a number or type -1 to end program: ")) if number == -1: break counter += 1 if number > largestNumber: largestNumber = number if counter != 0: print("The largest number is", largestNumber) else: print("You haven't entered any number.")
largestNumber = -99999999 counter = 0 number = int(input("Enter a number or type -1 to end program: ")) while number != -1: if number == -1: continue counter += 1 if number > largestNumber: largestNumber = number number = int(input("Enter a number or type -1 to end program: ")) if counter: print("The largest number is", largestNumber) else: print("You haven't entered any number.")
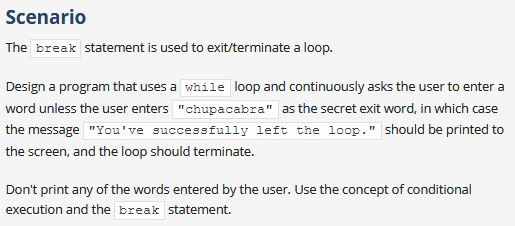
Using break
counter = 0 while True: word = input("Enter a word to end program: ") if word == "chupacabra": break counter += 1 if counter != 0: print("You've successfully left the loop.") else: print("You are genius.")
Now, the question is how to use continue to do it?
Here is an answer.
guessword = "chupacabra" counter = 0 word = input("Enter a word to end program: ") while word != "chupacabra": if word == "chupacabra": continue counter += 1 if word != guessword: guessword = word word = input("guess agian: ") if counter: print("You've successfully left the loop") else: print("You are a genius.")
Any other ways?
留言