Assignment Operators
- John Fan Zhang
- Jun 14, 2020
- 2 min read
Assignment operators are used in Python to assign values to variables. a = 5 is a simple assignment operator that assigns the value 5 on the right to the variable a on the left. There are various compound operators in Python like a += 5 that adds to the variable and later assigns the same. It is equivalent to a = a + 5.
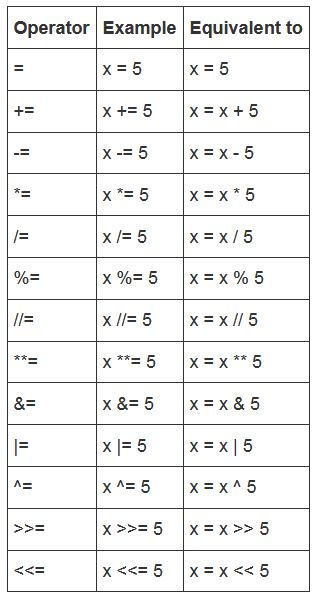
It is worth noting that:
**: exponentiation
^: exclusive-or (bitwise)
x << y Returns x with the bits shifted to the left by y places (and new bits on the right-hand-side are zeros). This is the same as multiplying x by 2**y.
x >> y Returns x with the bits shifted to the right by y places. This is the same as dividing x by 2**y.
Let's have a look at a short program whose task is to write some of the first powers of two:
pow = 1
for exp in range(16):
print("2 to the power of", exp, "is", pow)
pow *= 2
The exp variable is used as a control variable for the loop, and indicates the current value of the exponent. The exponentiation itself is replaced by multiplying by two.
Since 2**0 is equal to 1, then 2 × 1 is equal to 21, 2 × 21 is equal to 22, and so on.
In other words,
In the first round, "2 to the power of", 0, "is", 1
pow == pow * 2 == 1*2 = 2
In the second round, "2 to the power of", 1, "is", 2
pow == pow*2 == 2*2 = 4
In the third round, "2 to the power of", 1, "is", 4
pow == pow*4 == 4*2 = 8
...............................................................................
What is the greatest exponent for which our program still prints the result?
Answer:
2 to the power of 0 is 1
2 to the power of 1 is 2
2 to the power of 2 is 4
2 to the power of 3 is 8
2 to the power of 4 is 16
2 to the power of 5 is 32
2 to the power of 6 is 64
2 to the power of 7 is 128
2 to the power of 8 is 256
2 to the power of 9 is 512
2 to the power of 10 is 1024
2 to the power of 11 is 2048
2 to the power of 12 is 4096
2 to the power of 13 is 8192
2 to the power of 14 is 16384
2 to the power of 15 is 32768
Commenti