while i and for i in range ()
While Loop is used to repeat a block of code. Instead of running the code block once, It executes the code block multiple times until a certain condition is met.
For loop is used to iterate over elements of a sequence. It is often used when you have a piece of code which you want to repeat "n" number of time.
While loop does the exactly same thing what "if statement" does, but instead of running the code block once, they jump back to the point where it began the code and repeats the whole process again.
Imagine that a loop's body needs to be executed exactly one hundred times. If you would like to use the while loop to do it, it may look like this:
i = 0
while i < 100:
# do_something()
i += 1
In Python, "for loops" are called iterators. Unlike while loop which depends on condition true or false. "For Loop" depends on the elements it has to iterate.
for i in range(100):
print("The value of i is currently", i)
Run the code to check if you were right.
Note:
the loop has been executed ten times (it's the range() function's argument)
the last control variable's value is 99 (not 10, as it starts from 0, not from 1)
The range() function invocation may be equipped with two arguments, not just one:
for i in range(2, 8):
print("The value of i is currently", i)
Note:
The range() function accepts only integers as its arguments, and generates sequences of integers.
The first value shown is 2 (taken from the range()'s first argument.)
The last is 7 (although the range()'s second argument is 8).
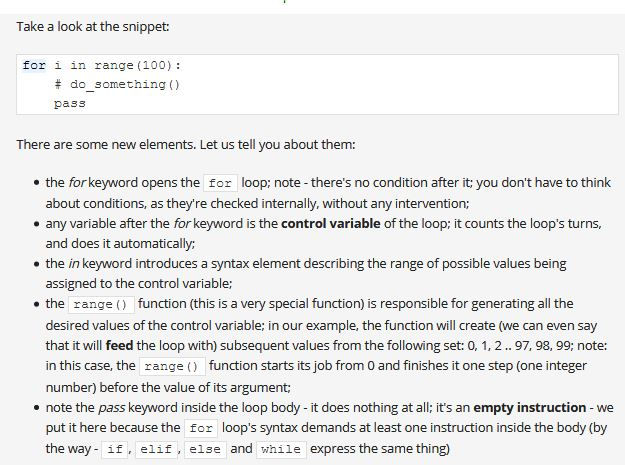
If we print(i), we will get number 99.
Good material: