Compact while()
while True means loop forever. The while statement takes an expression and executes the loop body while the expression evaluates to (boolean) "true". True always evaluates to boolean "true" and thus executes the loop body indefinitely. It's an idiom that you'll just get used to eventually! Most languages you're likely to encounter have equivalent idioms.
To terminate your program, just press Ctrl-C (or Ctrl-Break on some computers). This will cause the so-called KeyboardInterrupt exception and let your program get out of the loop. We'll talk about it later in the course.
Important:
if the condition is False (equal to zero) as early as when it is tested for the first time, the body is not executed even once (note the analogy of not having to do anything if there is nothing to do);
the body should be able to change the condition's value, because if the condition is True at the beginning, the body might run continuously to infinity - notice that doing a thing usually decreases the number of things to do).
How to code compactly for the following program?
# A program that reads a sequence of numbers # and counts how many numbers are even and how many are odd. # The program terminates when zero is entered. odd_numbers = 0 even_numbers = 0 # read the first number number = int(input("Enter a number or type 0 to stop: ")) # 0 terminates execution while number != 0: # check if the number is odd if number % 2 == 1: # increase the odd_numbers counter odd_numbers += 1 else: # increase the even_numbers counter even_numbers += 1 # read the next number number = int(input("Enter a number or type 0 to stop: ")) # print results print("Odd numbers count:", odd_numbers) print("Even numbers count:", even_numbers)
Note these two forms are equivalent:
while number != 0: and while number:.
The condition that checks if a number is odd can be coded in these equivalent forms, too:
if number % 2 == 1: and if number % 2:.
Because != 0 is true and ==1 is also true!!
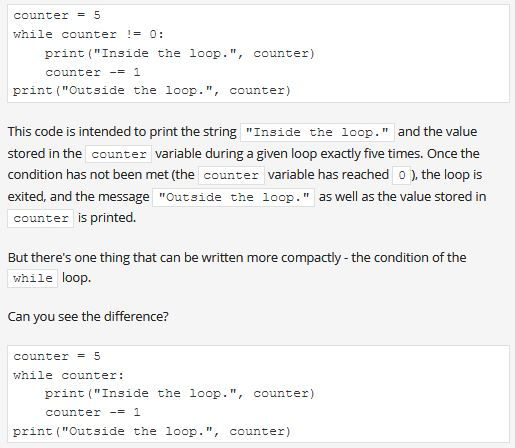