float() and round()
The float() method is used to return a floating point number from a number or a string.
Syntax: float(x)
The method only accepts one parameter and that is also optional to use. Let us look at the various types of argument, the method accepts:
A number : Can be an Integer or a floating point number.
A String :
Must contain numbers of any type.
Any left or right whitespaces or a new line is ignored by the method.
Mathematical Operators can be used.
Can contain NaN, Infinity or inf (any cases)
Values that the float() method can return depending upon the argument passed
If an argument is passed, then the equivalent floating point number is returned.
If no argument is passed then the method returns 0.0 .
If any string is passed that is not a decimal point number or does not match to any cases mentioned above then an error will be raised.
If a number is passed outside the range of Python float then OverflowError is generated.
The round() function returns a floating point number that is a rounded version of the specified number, with the specified number of decimals.
The default number of decimals is 0, meaning that the function will return the nearest integer.
Syntax: round(number, digits)
Parameter Values:
numberRequired. The number to be rounded
digitsOptional. The number of decimals to use when rounding the number. Default is 0
Scenario
Once upon a time there was a land - a land of milk and honey, inhabited by happy and prosperous people. The people paid taxes, of course - their happiness had limits. The most important tax, called the Personal Income Tax (PIT for short) had to be paid once a year, and was evaluated using the following rule:
if the citizen's income was not higher than 85,528 thalers, the tax was equal to 18% of the income minus 556 thalers and 2 cents (this was the so-called tax relief)
if the income was higher than this amount, the tax was equal to 14,839 thalers and 2 cents, plus 32% of the surplus over 85,528 thalers.
Your task is to write a tax calculator.
It should accept one floating-point value: the income.
Next, it should print the calculated tax, rounded to full thalers. There's a function named round() which will do the rounding for you - you'll find it in the skeleton code in the editor.
Note: this happy country never returns money to its citizens. If the calculated tax is less than zero, it only means no tax at all (the tax is equal to zero). Take this into consideration during your calculations.
income = float(input("Enter the annual income: "))
if income <= 85528.00: tax = (0.18 * income - 556.02) # Put your code here. else: tax = (14839.02 + 0.32 * (income - 85528.00))
if tax > 0.00: tax = round(tax, 0) else: tax = 0.00
print("The tax is:", tax, "thalers")
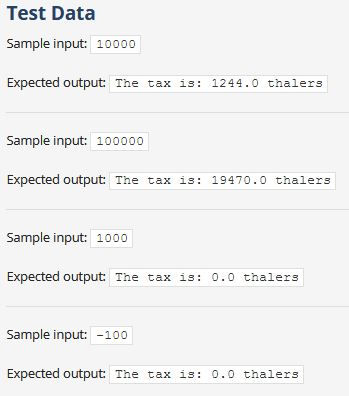
Note: As the input is float number, the programming definition should be consistent with it.