Time evaluation
- John Fan Zhang
- Jun 7, 2020
- 2 min read
Prepare a simple code able to evaluate the end time of a period of time, given as a number of minutes (it could be arbitrarily large). The start time is given as a pair of hours (0..23) and minutes (0..59). The result has to be printed to the console.
Key:
(hour + dura)//60 will give you the number of hours,
You'll also have to check the hours for %24 to make sure you roll the hours over.
(mins + dura)%60 will give you the number of minutes.
You'll also have to check the minutes for %60 to make sure you roll minutes over.
Example:
hour = int(input("Starting time (hours): ")) mins = int(input("Starting time (minutes): ")) dura = int(input("Event duration (minutes): "))
# find a total of all minutes
mins = mins + dura%60
# find a number of hours hidden in minutes and update the hour
hour = hour + dura//60 + mins//60
# correct minutes to fall in the (0..59) range mins = mins%60
# correct hours to fall in the (0..23) range hour = hour%24 print(hour, ":", mins, sep='')
This is the standard way to do these kinds of problems.
Division tells you how many times one number can fit inside another, like 20 // 3 = 6, so 3 can fit inside 20, 6 times.
Then modulo gives you the remainder of that operation. It's like if you kept doing 20 - 3, 17 - 3, 14 - 3, 11 - 3, 8 - 3, 5 - 3, then you get 2 and you're done, you can't take any more 3s away without going negative, so the remainder is 2. That's what the modulo gives you. 20%3 = 2.
So division and modulo are kinda 2 sides of the same coin here, the division handles the "big" part and modulo handles the "small" leftover.
Adding all the minutes and doing division will tell you have how many times around the clock you looped (hours), and then the modulo is the remainder, the minutes you're left at.
Here's an example: 17:52, duration: 8 hours 19 minutes
(499 minutes => 499%60 = 19 minutes)
Minutes: 52+19 = 71 => 71 % 60 = 11
Hours: 71 // 60 = 1 => 17 + (8 + 1) = 26 hours, 11 minutes.
You'll want to then do 26 % 24 for the final time 02:11.
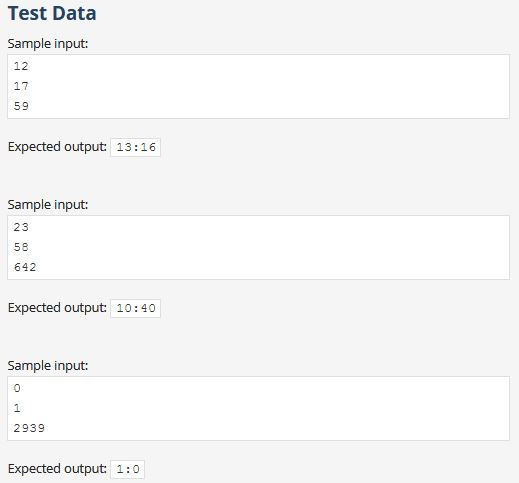
See a discussion here: https://www.reddit.com/r/learnpython/comments/ee7i5w/how_to_write_a_python_code_able_to_evaluate_end/
Comentarios