end=, sep=, +, *, and /
- John Fan Zhang
- Jun 6, 2020
- 2 min read
Python’s print() function comes with a parameter called ‘end’. By default, the value of this parameter is ‘\n’, i.e. the new line character. You can end a print statement with any character/string using this parameter. => https://www.geeksforgeeks.org/gfact-50-python-end-parameter-in-print
print("Welcome to" , end = ' ')
print("GeeksforGeeks", end = ' ')
Output => Welcome to GeeksforGeeks
print("Python" , end = '@')
print("GeeksforGeeks")
Output => Python@GeeksforGeeks
The separator between the arguments to print() function in Python is space by default (softspace feature) -- one space. The ‘sep=’ is used to achieve different types of spaces. Examples: #code for disabling the softspace feature print('G','F','G', sep='') #for formatting a date print('09','12','2016', sep='-') #another example print('pratik','geeksforgeeks', sep='@') Output: GFG 09-12-2016 pratik@geeksforgeeks
The 'sep=' used with 'end=' produces awesome results. print('G','F', sep='', end='') print('G') #\n provides new line after printing the year print('09','12', sep='-', end='-2016\n') print('prtk','agarwal', sep='', end='@') print('geeksforgeeks') Output: GFG 09-12-2016 prtkagarwal@geeksforgeeks
The + (plus) sign, when applied to two strings, becomes a concatenation operator: string + string Examples: fnam = input("May I have your first name, please? ") lnam = input("May I have your last name, please? ") print("Thank you.") print("\nYour name is " + fnam + " " + lnam + ".")
The last line is the same with print("\nYour name is", fnam, lnam + ".", sep=" ")
It is also the same with
print("\nYour name is", end=" ") print(fnam, lnam+".", sep=" ")
The * (asterisk) sign, when applied to a string and number (or a number and string, as it remains commutative in this position) becomes a replication operator: string * number or number * string
For example:
"James" * 3 gives "JamesJamesJames"
3 * "an" gives "ananan"
5 * "2" (or "2" * 5) gives "22222" (not 10!)
A number less than or equal to zero produces an empty string.
Try this simple program to "draws" a rectangle
print("+" + 10 * "-" + "+")
print(("|" + " " * 10 + "|\n") * 5, end="")
print("+" + 10 * "-" + "+")
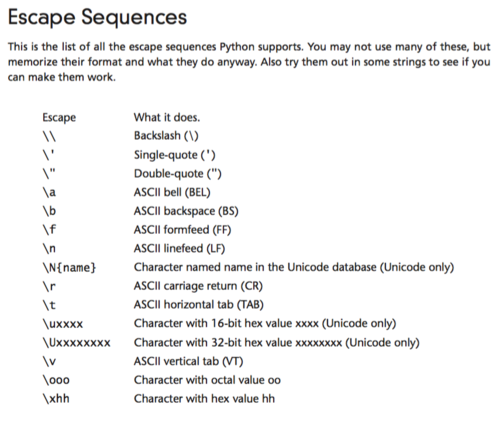
Comments